When a HubSpot website or landing page is visited by a known contact, you can pass the contact information into Mindstamp videos to achieve personalization and enhanced analytics:
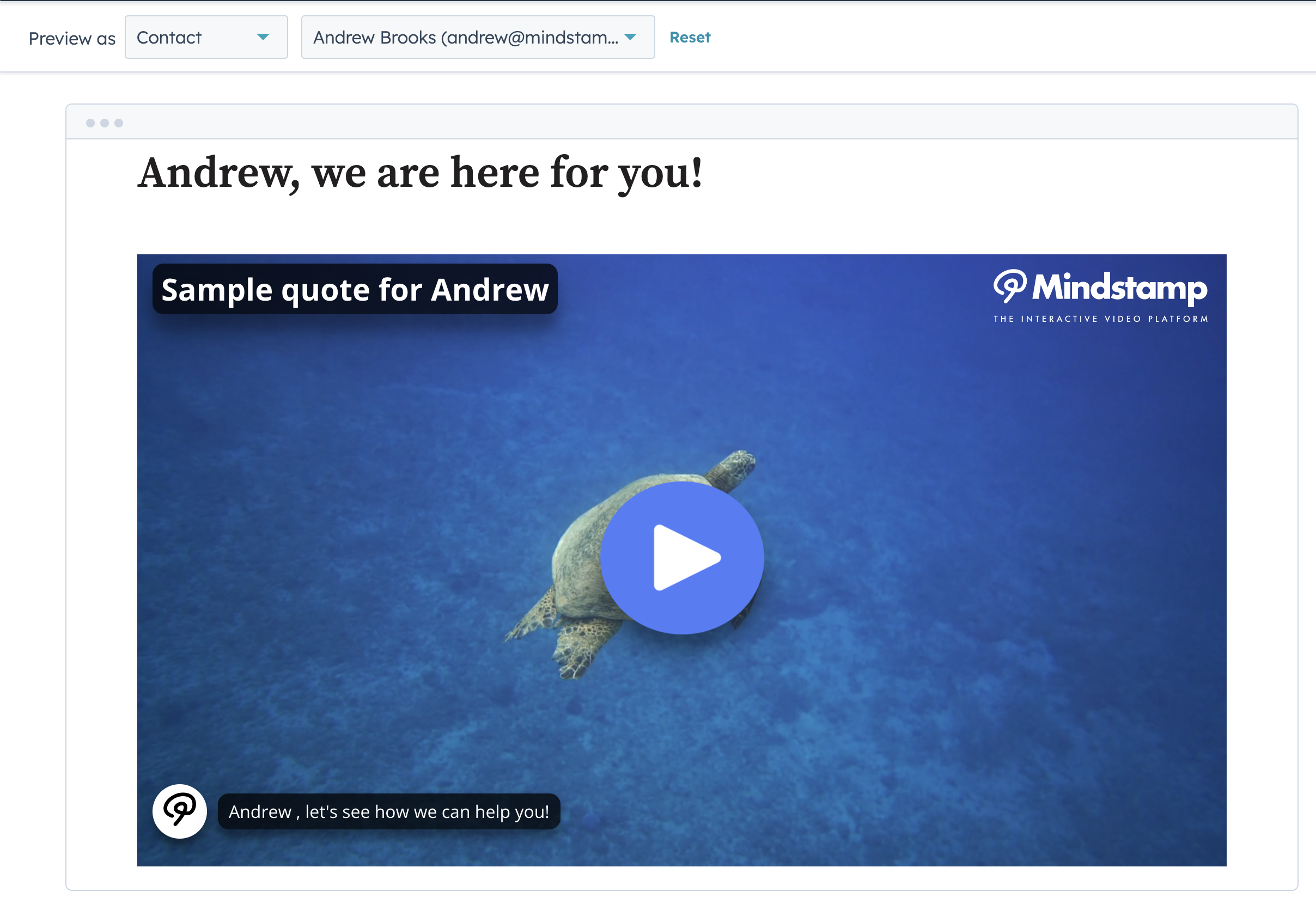
You can add the following code snippet to the footer of your HubSpot pages to achieve this personalization:
<div style="visibility: hidden;">
<span id="hubspot_name"> {{ contact.firstname }} </span>
<span id="hubspot_email"> {{ contact.email }} </span>
</div>
<script defer>
setTimeout(()=> {
// Set your data in these fields from the DOM or JS layer, e.g. set name to document.getElementById('viewer_name').innerHTML.trim()
var params = {
name: document.getElementById("hubspot_name").innerHTML,
email: document.getElementById("hubspot_email").innerHTML,
phone: "",
custom_id: "",
}
var queryString = Object.keys(params).map(function(key) {
return params[key] ? key + '=' + params[key] : ''
})
queryString = queryString.filter(Boolean).join("&")
if (queryString.length) {
frames = document.getElementsByTagName("iframe");
for (i = 0; i < frames.length; ++i) {
if (frames[i].src.includes("mindstamp") || frames[i].src.includes("myinteractive")) {
var url = frames[i].src + (frames[i].src.includes("?") ? "&"+queryString : "?"+queryString)
frames[i].src = url
}
}
}
})
</script>
That's it! Now you have personalized interactive videos on your HubSpot landing pages.
Technical Explanation:
The code above does two things: surfaces the contact's details, then passes them into the videos on the page.
First, we need to surface the data that you want to use within the landing page so that our personalization script can grab it and pass it to the video. This means using personalization tokens within your landing page and giving the element an ID that you will later use to retrieve it, like so:
<div style="visibility: hidden;">
<span id="hubspot_name"> {{ contact.firstname }} </span>
<span id="hubspot_email"> {{ contact.email }} </span>
</div>
This code surfaces the visitors name and email in a hidden block, so that it's on the page but is not visible to them.
The final step is to modify and include our personalization snippet such that it grabs the information you surfaced in step 1 and passes it to the video. Here is the snippet modified to grab the name and email information we are using in step 1:
<script defer>
setTimeout(()=> {
// Set your data in these fields from the DOM or JS layer, e.g. set name to document.getElementById('viewer_name').innerHTML.trim()
var params = {
name: document.getElementById("hubspot_name").innerHTML,
email: document.getElementById("hubspot_email").innerHTML,
phone: "",
custom_id: "",
}
var queryString = Object.keys(params).map(function(key) {
return params[key] ? key + '=' + params[key] : ''
})
queryString = queryString.filter(Boolean).join("&")
if (queryString.length) {
frames = document.getElementsByTagName("iframe");
for (i = 0; i < frames.length; ++i) {
if (frames[i].src.includes("mindstamp") || frames[i].src.includes("myinteractive")) {
var url = frames[i].src + (frames[i].src.includes("?") ? "&"+queryString : "?"+queryString)
frames[i].src = url
}
}
}
})
</script>
You can see that we've modified lines 5 and 6 to respectively grab the name and email on the page, based on the HTML we set up in step 1. You could expand this code to grab and pass any data fields on the HubSpot contact record that you want by following the same pattern.
Putting it all together, here is a snippet that you can place on your landing pages that automatically passes the contact's name and email to the video if the visitor is known:
<div style="visibility: hidden;">
<span id="hubspot_name"> {{ contact.firstname }} </span>
<span id="hubspot_email"> {{ contact.email }} </span>
</div>
<script defer>
setTimeout(()=> {
// Set your data in these fields from the DOM or JS layer, e.g. set name to document.getElementById('viewer_name').innerHTML.trim()
var params = {
name: document.getElementById("hubspot_name").innerHTML,
email: document.getElementById("hubspot_email").innerHTML,
phone: "",
custom_id: "",
}
var queryString = Object.keys(params).map(function(key) {
return params[key] ? key + '=' + params[key] : ''
})
queryString = queryString.filter(Boolean).join("&")
if (queryString.length) {
frames = document.getElementsByTagName("iframe");
for (i = 0; i < frames.length; ++i) {
if (frames[i].src.includes("mindstamp") || frames[i].src.includes("myinteractive")) {
var url = frames[i].src + (frames[i].src.includes("?") ? "&"+queryString : "?"+queryString)
frames[i].src = url
}
}
}
})
</script>
If you need help, get in touch.